C# 高级
发布时间 :
字数:1.1k
C# 高级
NameSpace
把框架写入命名空间中
System和System.Collections.Generic不是父子或包含关系 除非存在嵌套
同一个namespace的类名不能重复 不同间的可以
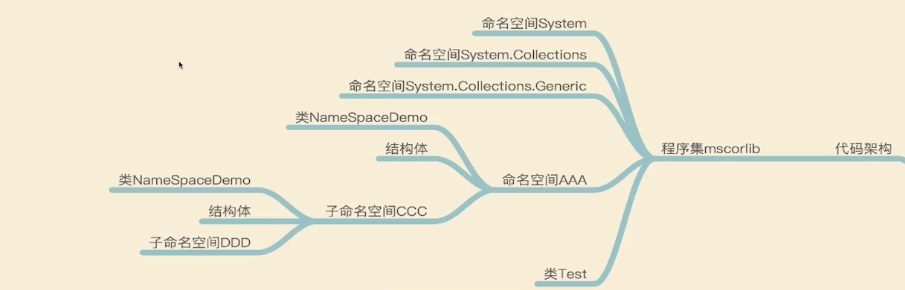
DLL文件为动态链接库文件,又称“应用程序拓展”,DLL文件中存放的是各类程序的函数(子过程)实现过程,当程序需要调用函数时需要先载入DLL,然后取得函数的地址,最后进行调用。
TryCatch
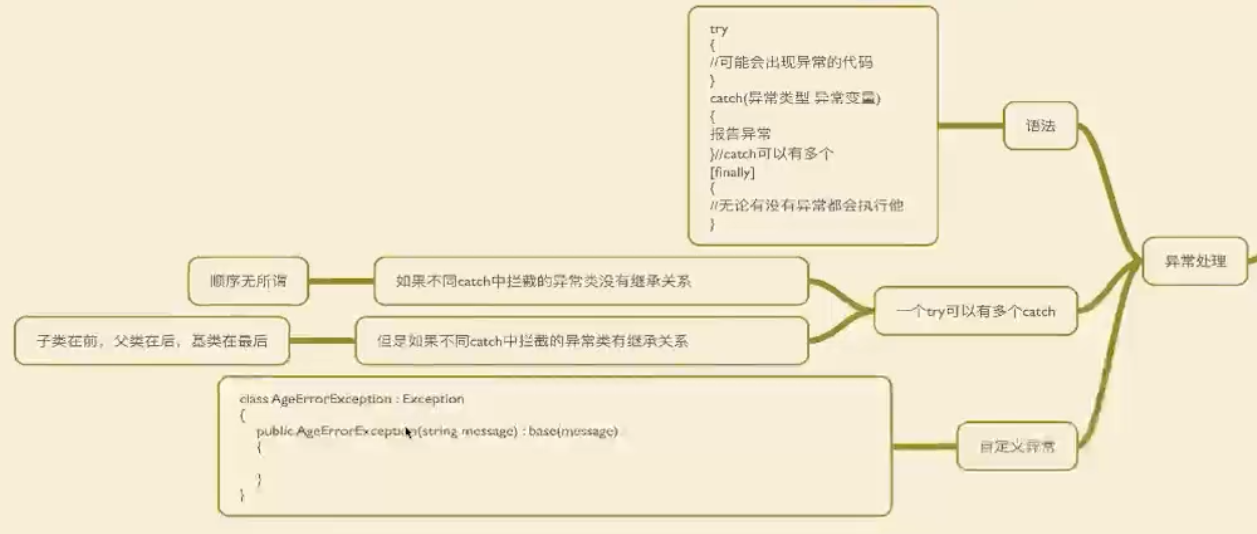
1 2 3 4 5 6
| try{ ... } catch(xxxException ex){ Debug.Log(); }
|
多个catch异常类型子类在前父类在后
自定义异常
由于用户操作(如不符合规定的输入)引发的异常
1 2 3 4 5 6 7 8
| public class xxxException : Exception{ public xxxException(string message):base(message){
} }
throw new xxxException("xxx异常");
|
ReflectionType
获取反射类型
typeof 通过类名
Type xxxType = typeof(xxx);
Type xxxType = typeof(namespace.xxx);//有命名空间的类
GetType() 通过对象
Type transformType = transform.GetType();
static GetType() 通过类名的字符串
Type xxxType = Type.GetType(namespace.xxx);
Type类中的属性
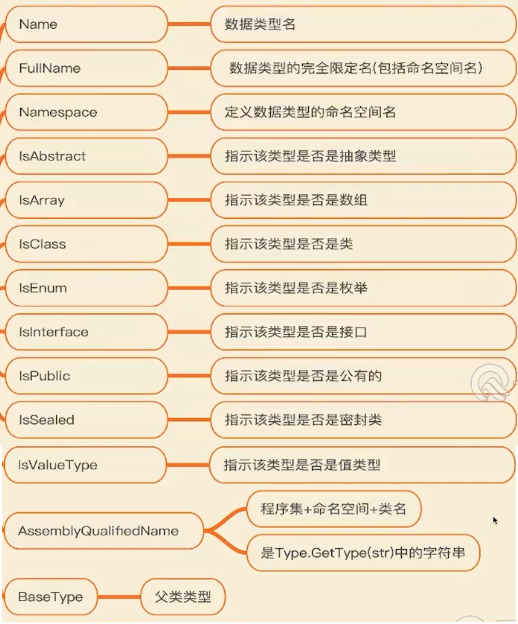
Type类中的方法
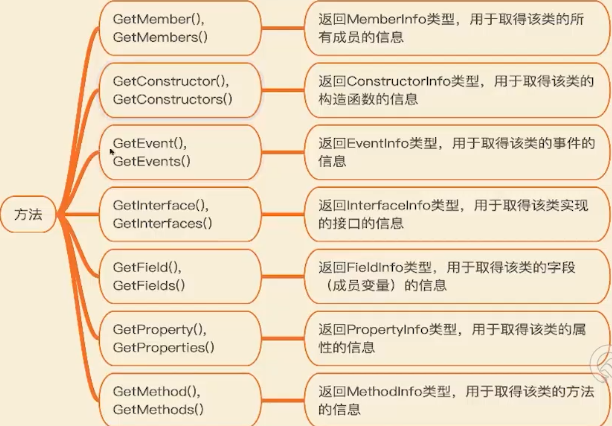
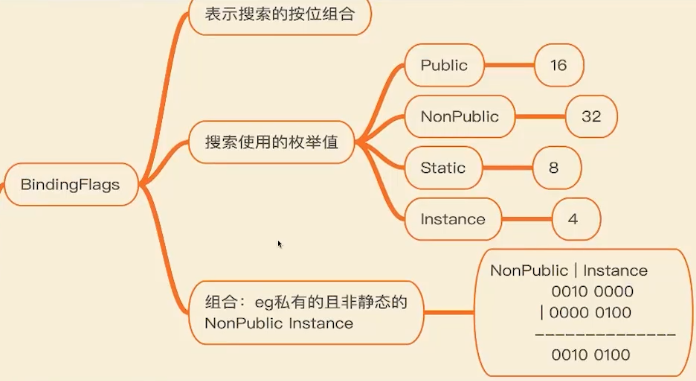
用类型实例化对象
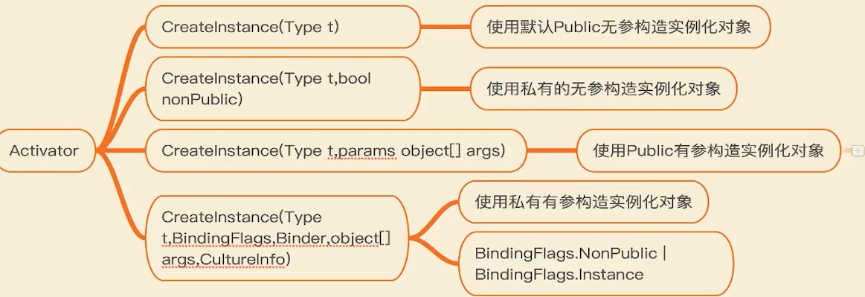
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
|
Type goType = typeof(GameObject);
object goObj = Activator.CreateInstance(goType);
(goObj as GameObject).AddComponent<ParticleSystem>();
Type singletonType = typeof(Singleton); object sgObj = Activator.CreateInstance(singletonType,true);
Debug.Log((sgObj as Singleton).name);
Type singletonType = typeof(Singleton);
object stObj = Activator.CreateInstance(singletonType, "laowang");
Debug.Log((stObj as Singleton).name);
Type singletonType = typeof(Singleton);
object obj = Activator.CreateInstance(singletonType, BindingFlags.Instance | BindingFlags.Public, null, new object[] { 12 }, null); Debug.Log((obj as Singleton).name); Debug.Log((obj as Singleton).age); obj = Activator.CreateInstance(singletonType, BindingFlags.Instance | BindingFlags.NonPublic, null, new object[] { "xiaomei", 12, "beijing" }, null); Debug.Log((obj as Singleton).name); Debug.Log((obj as Singleton).age); Debug.Log((obj as Singleton).address);
|
通过反射访问字段
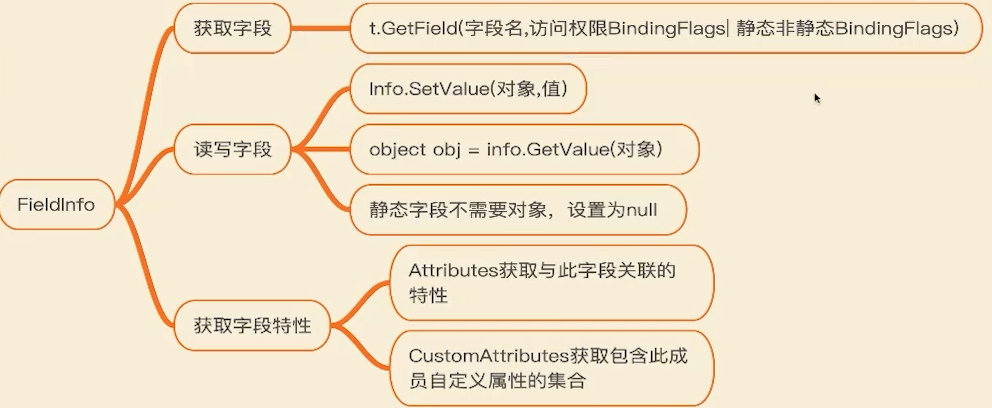
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| Type sgType = typeof(Singleton);
FieldInfo nameField = sgType.GetField("name");
object sgObj = Activator.CreateInstance(sgType, true);
nameField.SetValue(sgObj, "胖枫");
object nameValue = nameField.GetValue(sgObj);
Debug.Log(nameValue);
FieldInfo moneyField = sgType.GetField("money", BindingFlags.Instance | BindingFlags.NonPublic);
moneyField.SetValue(sgObj, 9999); (sgObj as Singleton).GetMoney();
|
通过反射访问方法
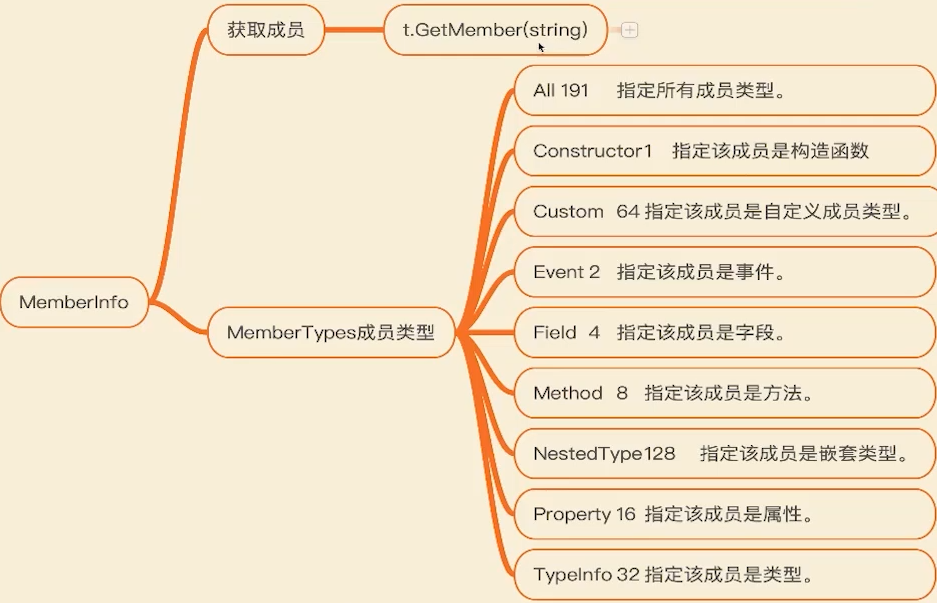
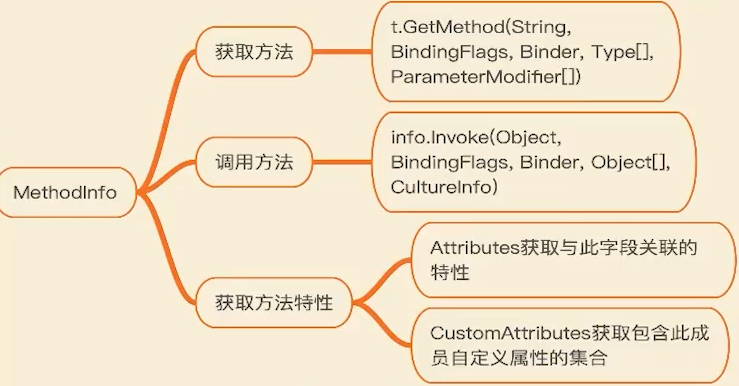
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| Type sgType = typeof(Singleton);
MethodInfo setMoneyMethod = sgType.GetMethod("SetMoney", BindingFlags.Instance | BindingFlags.NonPublic);
setMoneyMethod.Invoke(Singleton.GetInstance(), new object[] { 8888 }); Singleton.GetInstance().GetMoney();
MethodInfo setmoneyMethod = sgType.GetMethod("SetMoney", BindingFlags.Instance | BindingFlags.NonPublic, null, new Type[] { typeof(string) }, null);
setmoneyMethod.Invoke(Singleton.GetInstance(), new object[] { "8888" }); Singleton.GetInstance().GetMoney();
MethodInfo setmoneyMethod = sgType.GetMethod("SetMoney", BindingFlags.Instance | BindingFlags.NonPublic, null, new Type[] { typeof(string), typeof(float) }, null);
setmoneyMethod.Invoke(Singleton.GetInstance(), new object[] { "8888", 2 }); Singleton.GetInstance().GetMoney();
|
批量调用方法
{“methods”:[“A”,”B”,”C”]}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| string[] methods = new string[] { "SetName","SetMoney","SetEquips" }; for (int i = 0; i < methods.Length; i++) {
try { InvokeMethod(methods[i], new Type[] { typeof(string), typeof(float) }, new object[] { "8888", 2 }); } catch (Exception ex) {
} } public void InvokeMethod(string methodName,Type[] types,object[] realParams) { Type sgType = typeof(Singleton); MethodInfo setmoneyMethod = sgType.GetMethod(methodName, BindingFlags.Instance | BindingFlags.NonPublic, null, types, null); setmoneyMethod.Invoke(Singleton.GetInstance(), realParams); Singleton.GetInstance().GetMoney(); }
|
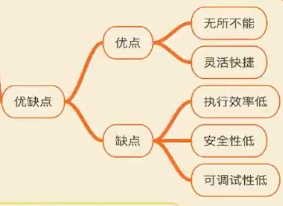
Attrubutes
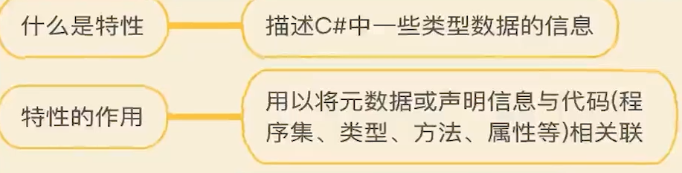
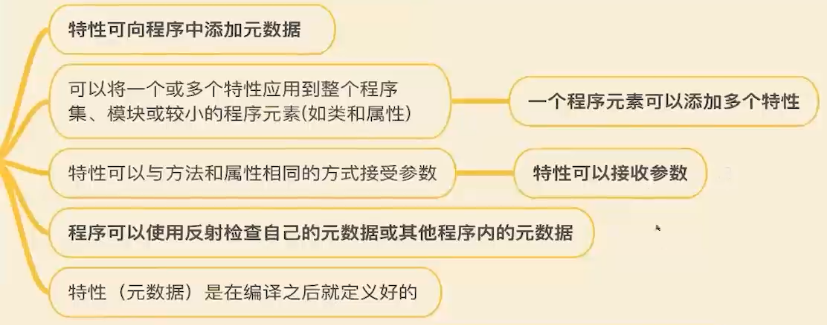
sort
.Net
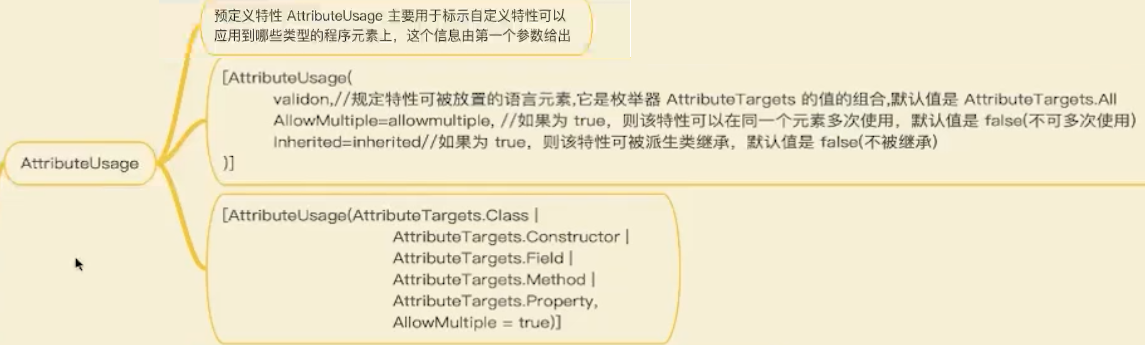
1 2 3 4 5 6 7 8 9 10 11 12 13
| [AttributeUsage(AttributeTargets.All,AllowMultiple = true)] public class AuthorAttribute : Attribute { public string authorName; public char authorSex; public string datatime; public AuthorAttribute(string authorName,char authorSex,string datatime) { this.authorName = authorName; this.authorSex = authorSex; this.datatime = datatime; } }
|
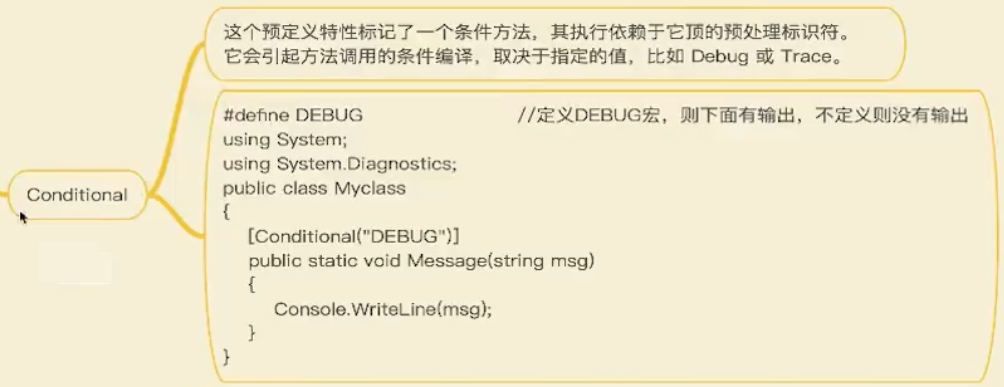
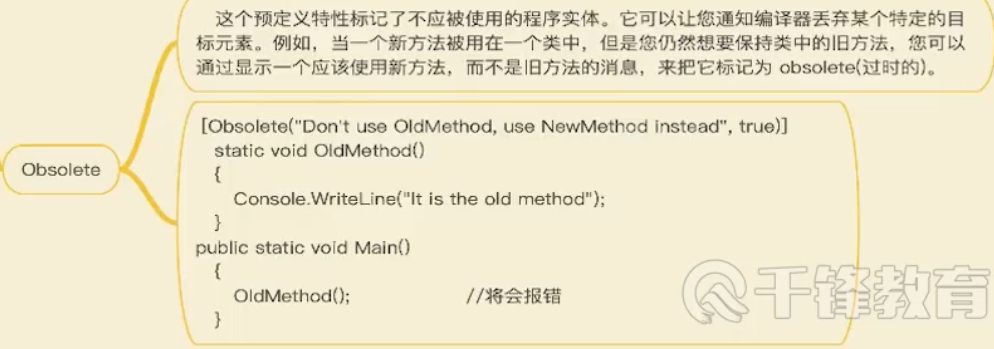
第二个参数为true则旧方法会报错
customize
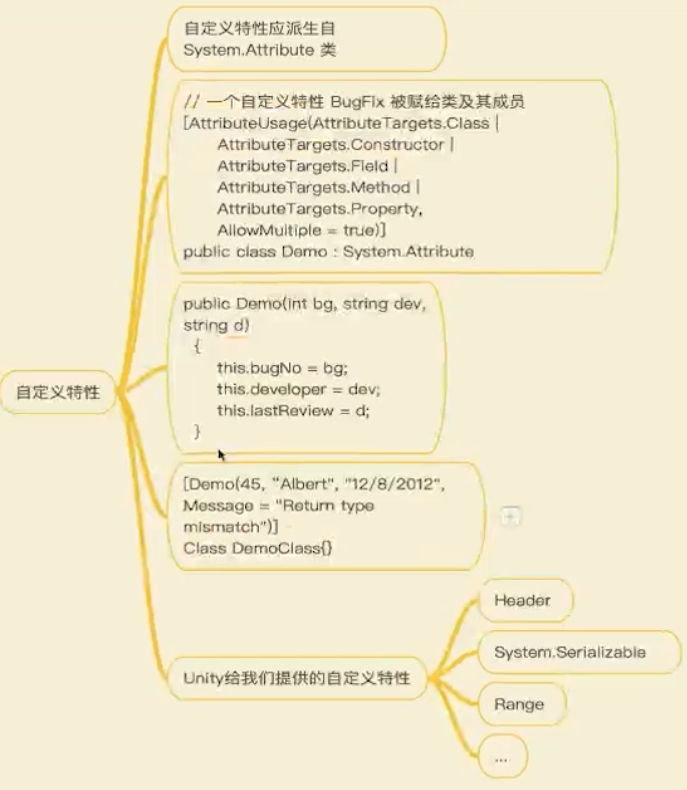
用反射展示某个类型的信息
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| ShowMemberAuthorMsg(gameObject.GetType()); MemberInfo[] memberInfo = this.GetType().GetMembers(); for (int i = 0; i < memberInfo.Length; i++) ShowMemberAuthorMsg(memberInfo[i]); public void ShowMemberAuthorMsg(MemberInfo memberInfo) { object[] atts = memberInfo.GetCustomAttributes(false); for (int i = atts.Length - 1; i >= 0; i--) { if (atts[i] is AuthorAttribute) { AuthorAttribute author = atts[i] as AuthorAttribute; } } }
methodInfo.GetCustomAttributes(true)
|
正则表达式